Quick Example
Dockerfile:
FROM python:3.8-slim-buster
RUN python -m pip install fire
Build image:
docker build --tag python-docker .
Mount host machine path (scripts) to python project and execute scripts/test.py from container:
docker run -v /mnt/d/temp/dockertests/python3/scripts/:/scripts python-docker python /scripts/test.py
Detailed Explanations
First step is to install Docker on your platform. You can follow the steps at How To Setup WordPress on Docker WSL For Development On A Local Directory or the official guide at https://docs.docker.com/get-docker/
Create a Dockerfile
Create a folder, for example one called python, and then inside the folder create a file named Dockerfile. Inside the Dockerfile insert the following contents:
FROM python:3.8-slim-buster
This is will instruct docker on docker build to fetch the official python image from Dockerhub and also here we are asking docker to pull the slim version which only has the common packages for python3.8.
How to build your own Python Docker Image?
You can build the image by going to the folder you where the Dockerfile above is present and running the following command:
docker build --tag python-docker .
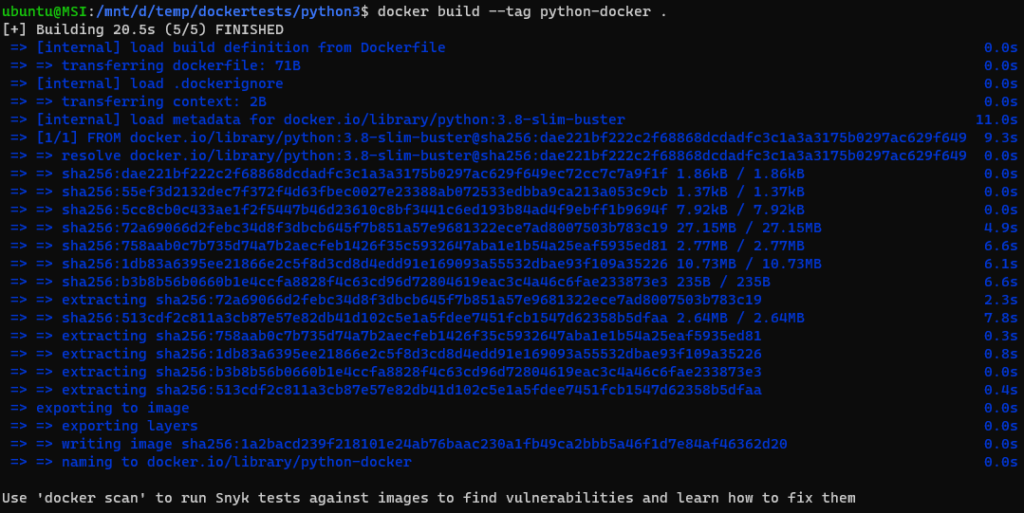
We are telling docker to build the image in the current folder . and to give a tag: python-docker. We can now use python-docker to run python3.8. For example:
docker run -it --rm python-docker
This tells docker to the image we just created.
What does Docker run -it do?
We are telling docker to run the container in Interactive mode with -i and we are telling docker to put the output in our terminal with -t.
What does Docker –rm do?
–rm tell Docker to delete the container after running.
Running the above command should give an interactive shell of python3.8.

How to run a python script with Docker?
If you want to run a script you need to point the container to that script. This can be done either by putting the script inside the container or by mounting the location where the script is on your host machine and then pointing the container to run that script/s.
You can mount a location by providing the volume parameter -v. In the example the path I am currently at is /mnt/d/temp/dockertests/python3. Let’s say I create a test script test.py in that folder as follows:

To run the following script you can do the following:
docker run -v /mnt/d/temp/dockertests/python3/scripts/:/scripts python-docker python /scripts/test.py
What the above is doing is that it is mounting my host machine’s path /mnt/d/temp/dockertests/python3/scripts to /scripts (a new path) on the container. This gives the container access to everything that is in my host machine’s /mnt/d/temp/dockertests/python3/scripts path.
Then my container python-docker is called to execute “python /scripts/test.py” which is exactly how you would call it if you didn’t use Docker.
Note you should
How To Install Python PIP Modules On Docker?
Let’s say we change our script to now import fire. If you executed it would give you something like:
ubuntu@MSI:/mnt/d/temp/dockertests/python3$ docker run -v /mnt/d/temp/dockertests/python3/scripts/:/scripts python-docker python /scripts/test.py
Traceback (most recent call last):
File "/scripts/test.py", line 1, in <module>
import fire
ModuleNotFoundError: No module named 'fire'
You will need to add the pip install fire command to the Dockerfile to make sure the container imports it too. You can do that with:
FROM python:3.8-slim-buster
RUN python -m pip install fire
Your Dockerfile now also tells Docker to install the pip module fire.
You will now need to rebuild your container using:
docker build --tag python-docker .
You should now see Docker installing the module during building:
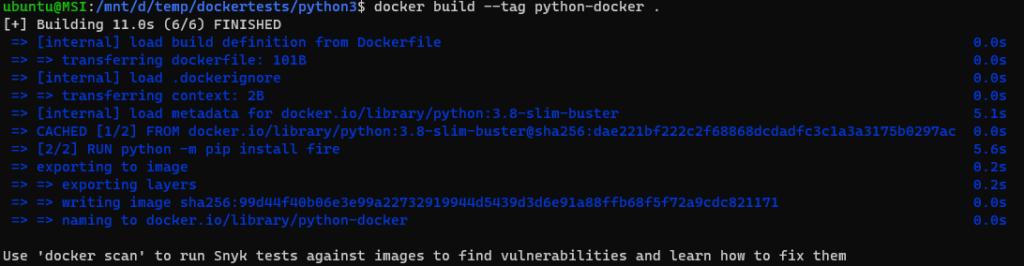
You should now be able to run your scripts, projects and install any dependencies you want. The above works both python2 and python3 just modify your Dockerfile to get version 2!
Happy coding 🙂
If you would like to learn more Python check out this certificate course by Google: IT Automation with Python